Eureka 找到啦!
服务注册与发现
简介
- Spring Cloud 封装了 Netflix 公司开发的 Eureka 模块来实现服务注册和发现。采用了 C-S 的设计架构。用来简化与服务器的交互、作为轮询负载均衡器,并提供服务的故障切换支持。
- 由两个组件组成。
- Ereka Server。 注册中心
- Ereka Client。 服务注册
Eureka 采用了客户端发现的方式,在服务运行时,通过(轮训、hash等负载均衡机制等方式)注册中心找到需要服务(即 A 通过 注册中心 找 B,需要谁找谁)。
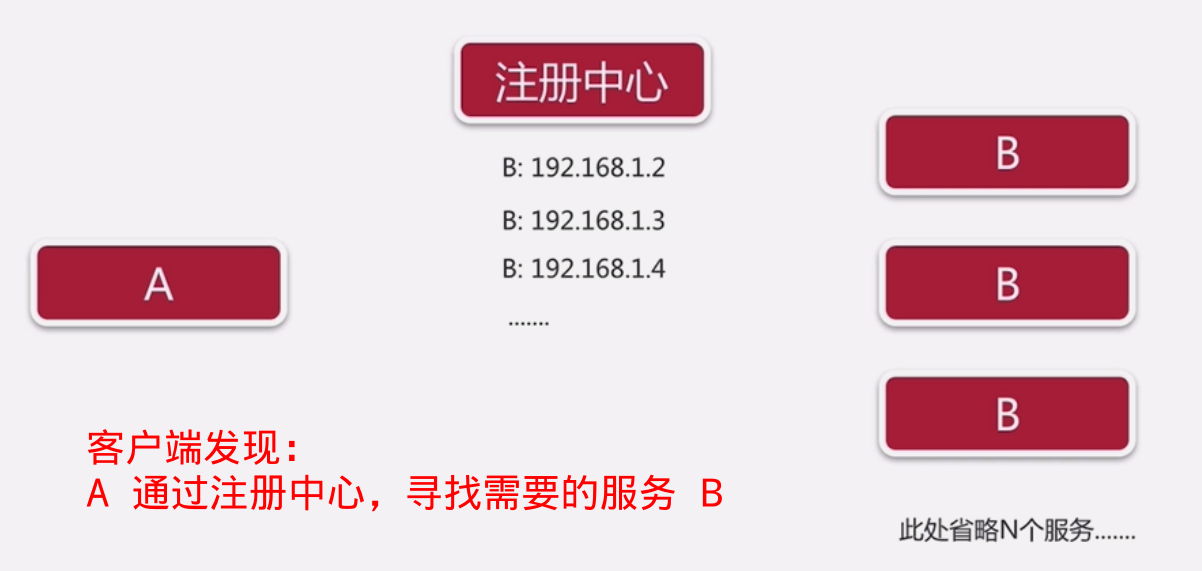
Eureka Server
注册中心记录着所有应用的信息和状态(如:应用名,所在服务器,是否正常工作)。
实现一个注册中心
1. 引入依赖
1
2
3
4
5
6
7
8
9
10
| <dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka-server</artifactId>
</dependency>
</dependencies>
|
2. 添加启动注解
1
2
3
4
5
6
7
| @EnableEurekaServer
@SpringBootApplication
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
|
3. 配置文件
1
2
3
4
5
6
7
8
9
10
11
12
13
| spring:
application:
name: eureka-server
Server:
port: 8761
eureka:
client:
register-with-eureka: false # 是否将自己注册到Eureka Server,作为 Server 端不需要
fetch-registry: false # 是否从Eureka Server获取注册信息,作为 Server 端不需要
service-url: # 接收的是一个 Map 结构
defaultZone: http://localhost:8761/eureka/
|
4. 启动程序
启动后,访问 http://localhost:8761/,即可看到 Spring Eureka 界面。
实现 Eureka 集群
在一个分布式系统中,服务注册中心是最重要的基础部分,理应随时处于可以提供服务的状态。为了维持其可用性,通常会采用集群的方案。Eureka通过互相注册的方式来实现高可用的部署
双节点注册
创建两台服务器,端口分别为 8761 和 8762。
将 8761 的服务器配置指向 8761,将 8762 的服务器指向 8761。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
| spring:
application:
name: eureka-server
Server:
port: 8761
eureka:
client:
register-with-eureka: false
fetch-registry: false
service-url: # 将 service-url 指向 8762
defaultZone: http://localhost:8762/eureka/
---
spring:
application:
name: eureka-server
Server:
port: 8762
eureka:
client:
register-with-eureka: false
fetch-registry: false
service-url: # 将 service-url 指向 8761
defaultZone: http://localhost:8761/eureka/
|
启动程序后,通过 http://localhost:8761/ 和 http://localhost:8762/ 都可访问 Eureka 界面。并且可以看到另一个节点信息节点的信息。
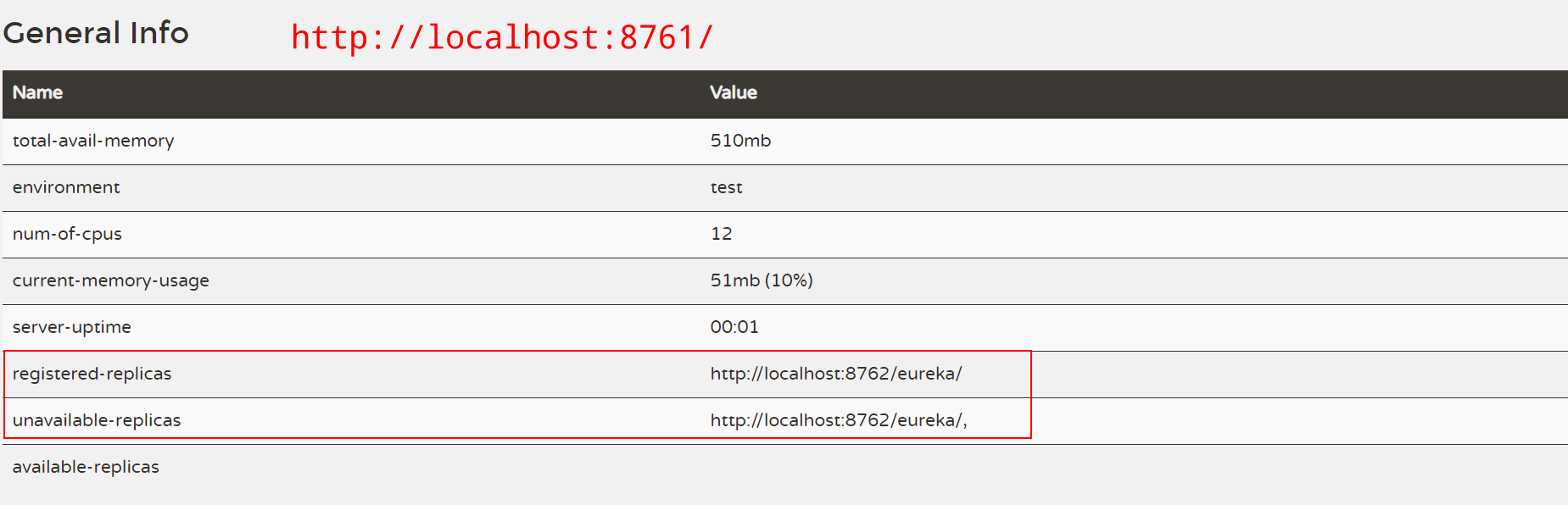
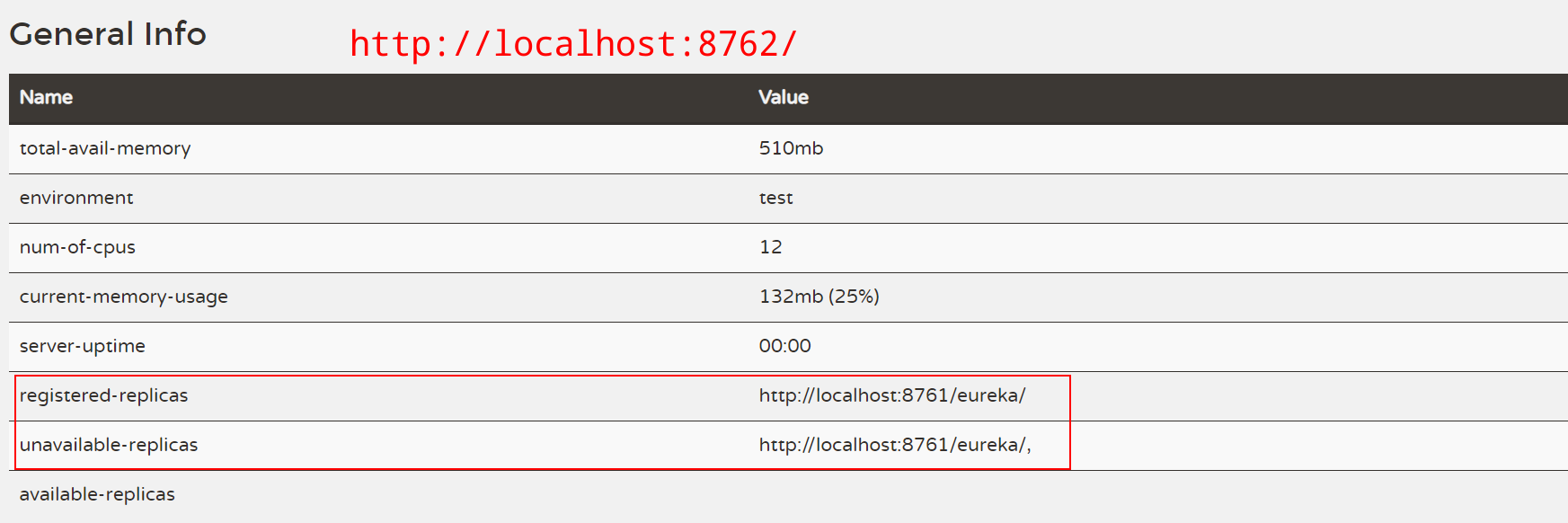
多节点注册
在生产中我们需要三台或者大于三台的注册中心来保证服务的稳定性,配置的原理其实都一样:将注册中心分别指向其它的注册中心。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
| ---
spring:
application:
name: eureka-server
server:
port: 8761
eureka:
client:
register-with-eureka: false
fetch-registry: false
serviceUrl:
defaultZone: http://localhost:8762/eureka/,http://localhost:8763/eureka/
---
spring:
application:
name: eureka-server
server:
port: 8762
eureka:
client:
register-with-eureka: false
fetch-registry: false
serviceUrl:
defaultZone: http://localhost:8761/eureka/,http://localhost:8763/eureka/
---
spring:
application:
name: eureka-server
server:
port: 8763
eureka:
client:
register-with-eureka: false
fetch-registry: false
serviceUrl:
defaultZone: http://localhost:8761/eureka/,http://localhost:8762/eureka/
|
Eureka Client
实现一个服务注册
1. 依赖配置
1
2
3
4
5
6
| <dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
</dependencies>
|
2. 添加启动注解
1
2
3
4
5
6
7
8
9
| import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@EnableDiscoveryClient
@SpringBootApplication
public class ClientApplication {
public static void main(String[] args) {
SpringApplication.run(ClientApplication.class, args);
}
}
|
3. 配置文件
1
2
3
4
5
6
7
8
9
10
11
12
13
| spring:
application:
name: eureka-client
server:
port: 8080
eureka:
client:
serviceUrl:
defaultZone: http://localhost:8761/eureka/
# instance: # 自定义链接
# hostname: example.com
|
4. 启动程序
启动程序后,进入 Eureka 页面 http://localhost:8761/eureka/,即可看到注册的服务 eureka-client。

总结
- 分布式系统中,服务注册中心是最重要的基础部分
- @EnableEurekaServer @EnableEurekaClient
- 具有 心跳检测,健康检查,负载均衡等功能
- 为保证高可用,建议集群部署